Introduction
Welcome to the heart of React development Props in React, where the concept of “props” transforms ordinary components into dynamic, interactive powerhouses. In the realm of React, understanding and mastering props is akin to wielding a mighty tool that empowers you to build flexible and reusable user interfaces. In this comprehensive guide, we will unravel the intricacies of props in React, shedding light on their significance, practical applications, and advanced techniques. Whether you’re a React enthusiast seeking to enhance your skills or a newcomer eager to harness the full potential of this JavaScript library, join us on this journey to unravel the secrets of props in React.
Table of Contents
Props in React
Understanding Props
1.1 What are Props?
Props, short for properties, are a crucial concept in React, a popular JavaScript library for building user interfaces. They provide a way to pass data from a parent component to its child components. This mechanism facilitates the creation of dynamic and reusable components within a React application.
In React, components are the building blocks of a user interface, representing different parts of the UI as modular and encapsulated entities. Props serve as a means of communication between these components, allowing them to exchange information and respond to changes in data.
When a parent component renders a child component, it can pass data to the child component through props. These props are essentially parameters that the child component can use to customize its behavior, appearance, or any other aspect determined by the parent.
1.2 Why Use Props?
The utilization of props in React brings forth several advantages that contribute to the overall efficiency and flexibility of a React application:
- Component Reusability: Props enable the creation of reusable components. By passing different sets of data through props, a single child component can adapt its behavior and appearance, making it versatile and reusable across various parts of the application.
- Maintainability: Props enhance code maintainability by establishing a clear and organized flow of data between components. With well-defined props, it becomes easier to understand how data is passed and used throughout the application, making future modifications and debugging more straightforward.
- Dynamic User Interfaces: Props allow for the creation of dynamic and interactive user interfaces. As the parent component provides updated data to its child components through props, the UI can dynamically respond to changes, providing users with a seamless and engaging experience.
- Communication Between Components: In a React application, different components may need to share information. Props serve as the communication bridge between these components, allowing them to exchange data without directly modifying each other’s internal state.
In summary, props play a vital role in React development by promoting reusability, maintainability, and dynamic user interfaces. Understanding how to effectively use props empowers developers to build scalable and flexible applications. This chapter will delve deeper into the practical implementation of props and explore various scenarios where they prove invaluable in React development.
Passing Props
2.1 Basic Prop Passing
In React, passing props from a parent component to a child component is a fundamental aspect of building modular and reusable user interfaces. The process involves providing information or parameters to a child component when it is rendered by its parent. This establishes a clear flow of data within the React component architecture.
Basic Prop Passing:
- Parent Component: When rendering a child component, you can pass props by including attributes within the component tag. For example:
// ParentComponent.jsx
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const dataToPass = "Hello from Parent!";
return (
<div>
<ChildComponent message={dataToPass} />
</div>
);
};
export default ParentComponent;
Child Component: The child component then receives the data through props and can use it accordingly.
// ChildComponent.jsx
import React from 'react';
const ChildComponent = (props) => {
return (
<div>
<p>{props.message}</p>
</div>
);
};
export default ChildComponent;
This basic example illustrates the flow of data from the parent component to the child component through props.
2.2 Prop Types
While passing props is a powerful feature, it’s equally important to ensure that the received data meets the expected requirements. Prop types provide a mechanism for enforcing data validation, catching potential bugs early in the development process, and improving code readability.
Prop Types:
- Install Prop Types: First, install the prop-types library:
npm install prop-types
Define Prop Types: Next, define prop types for your components, specifying the expected data types for each prop.
// ChildComponent.jsx
import React from 'react';
import PropTypes from 'prop-types';
const ChildComponent = (props) => {
return (
<div>
<p>{props.message}</p>
</div>
);
};
ChildComponent.propTypes = {
message: PropTypes.string.isRequired,
};
export default ChildComponent;
By defining prop types, you not only document the expected data structure but also enable React to issue warnings in development mode if the passed data does not adhere to the defined types. This proactive approach helps catch potential issues early in the development process, ensuring a more robust and error-resistant codebase.
This chapter has provided a foundational understanding of basic prop passing in React and emphasized the significance of prop types in maintaining data integrity within your components. In the following chapters, we’ll explore advanced techniques and best practices for leveraging props in various scenarios.
Destructuring Props
Destructuring is a powerful feature in JavaScript that allows you to extract values from objects and arrays, providing a concise and expressive way to work with data. In React, destructuring can be particularly beneficial when handling props in functional components, offering improved readability and a cleaner code structure.
3.1 Basic Destructuring:
Destructuring props simplifies the process of extracting values from the props
object, making the component code more concise and focused.
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ message }) => {
return (
<div>
<p>{message}</p>
</div>
);
};
export default ChildComponent;
Here, instead of using props.message
, the message
property is directly extracted from props
within the function parameters.
3.2 Default Values and Renaming:
Destructuring also allows you to set default values for props and rename variables during extraction.
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ message = "Default Message", author: sender }) => {
return (
<div>
<p>{message} from {sender}</p>
</div>
);
};
export default ChildComponent;
In this example, if the message
prop is not provided, it defaults to “Default Message.” Additionally, the author
prop is renamed to sender
during destructuring for improved clarity.
3.3 Destructuring in Function Parameters:
Destructuring can also be applied directly in the function parameters, making the component code more concise.
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ message, author }) => {
return (
<div>
<p>{message} from {author}</p>
</div>
);
};
export default ChildComponent;
This approach is especially useful when a component receives multiple props, as it allows for a clean and readable parameter list.
3.4 Nested Destructuring:
When dealing with nested objects or complex data structures, destructuring can be nested to extract values at different levels.
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ data: { message, author } }) => {
return (
<div>
<p>{message} from {author}</p>
</div>
);
};
export default ChildComponent;
Here, the data
prop is expected to be an object with message
and author
properties, and they are destructured directly within the function parameters.
By leveraging destructuring in your React components, you can enhance code readability, reduce redundancy, and create more expressive and concise functional components. This chapter has introduced the basics of destructuring props, showcasing its various applications in simplifying component code. In the upcoming chapters, we’ll delve into advanced techniques and explore real-world scenarios where destructuring props proves invaluable.
Default Props
In React, setting default values for props is a useful technique to ensure that your components gracefully handle scenarios where certain props are missing or undefined. Default props provide a fallback mechanism, allowing your components to maintain functionality even when specific data is not explicitly provided.
4.1 Setting Default Props:
To define default props for a component, you can use the defaultProps
property.
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ message, author }) => {
return (
<div>
<p>{message} from {author}</p>
</div>
);
};
ChildComponent.defaultProps = {
message: "Default Message",
author: "Anonymous",
};
export default ChildComponent;
In this example, if the message
or author
props are not provided when the component is used, the default values “Default Message” and “Anonymous” will be used, respectively.
4.2 Overriding Default Props:
It’s important to note that default props are overridden if a value is explicitly passed for the corresponding prop when rendering the component.
// ParentComponent.jsx
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const customMessage = "Custom Message";
const customAuthor = "John Doe";
return (
<div>
{/* Custom values provided, overriding defaults */}
<ChildComponent message={customMessage} author={customAuthor} />
{/* Defaults used for unspecified props */}
<ChildComponent />
</div>
);
};
export default ParentComponent;
In this example, when the ChildComponent
is rendered with custom values for message
and author
, the default values specified in defaultProps
are overridden. If the component is rendered without providing values for these props, the default values are used.
4.3 Use Cases for Default Props:
Setting default props is beneficial in various scenarios:
- Fallback Values: Ensure that your components always have sensible default values, preventing unexpected behavior if certain props are not provided.
- Increased Flexibility: Components with default props can be used with minimal configuration, simplifying their integration into different parts of your application.
- Code Documentation: Default props serve as documentation, providing insight into the expected behavior of a component when specific props are not provided.
By incorporating default props into your React components, you enhance their robustness and make them more adaptable to different usage scenarios. This chapter has introduced the concept of default props and demonstrated how to implement them effectively. In the following chapters, we’ll explore advanced techniques and best practices for handling props in React components.
Prop Drilling and Context API
5.1 Prop Drilling
In React applications, prop drilling refers to the process of passing props through multiple layers of components to reach a deeply nested child component. While prop drilling is a common pattern, it can lead to challenges in terms of code maintainability and readability.
5.1.1 Challenges of Prop Drilling:
- Complexity: As components become nested, passing props through each level can make the code complex and harder to understand.
- Maintenance: Modifying the structure of components or adding new props may require changes at multiple levels, making maintenance cumbersome.
- Code Bloat: Components in the middle of the hierarchy might receive props they don’t use but have to pass them down, contributing to code bloat.
5.1.2 Techniques to Mitigate Prop Drilling:
- Component Restructuring: Reorganize components to reduce the number of levels props need to traverse. This may involve creating new intermediate components or consolidating functionality.
- State Lifting: Elevate state to a common ancestor of components that need access to the state. This allows child components to receive the necessary data without prop drilling.
- Higher-Order Components (HOCs): Use HOCs to wrap components and inject the required props. This can help avoid prop drilling by providing the necessary props directly to the wrapped components.
5.2 Context API
The Context API is a powerful feature in React that addresses the challenges of prop drilling by providing a way to share values (such as state or functions) across components without passing props manually through each level.
5.2.1 Creating a Context:
// MyContext.js
import { createContext } from 'react';
const MyContext = createContext();
export default MyContext;
5.2.2 Providing Context at the Top Level:
Wrap the root component or a specific ancestor with the MyContext.Provider
and provide the values you want to share.
// App.js
import React from 'react';
import MyContext from './MyContext';
import ParentComponent from './ParentComponent';
const App = () => {
const sharedValue = "Shared Value";
return (
<MyContext.Provider value={sharedValue}>
<ParentComponent />
</MyContext.Provider>
);
};
export default App;
5.2.3 Consuming Context in a Child Component:
Access the shared value in any component by using the MyContext.Consumer
or the useContext
hook.
// ChildComponent.jsx
import React, { useContext } from 'react';
import MyContext from './MyContext';
const ChildComponent = () => {
const sharedValue = useContext(MyContext);
return (
<div>
<p>{sharedValue}</p>
</div>
);
};
export default ChildComponent;
5.2.4 Benefits of Context API:
- Simplified Data Sharing: Context API simplifies the process of sharing data between components, eliminating the need for prop drilling.
- Cleaner Code: Components can focus on their specific responsibilities without the clutter of passing irrelevant props.
- Centralized State: Centralize the state management in a single context, making it easier to manage and update shared values.
In summary, while prop drilling is a common pattern, it may lead to challenges in large and complex applications. The Context API provides an elegant solution to these challenges, offering a clean and efficient way to share values across components. This chapter has explored techniques to mitigate prop drilling and introduced the Context API as a powerful alternative. In the upcoming chapters, we’ll delve deeper into advanced use cases and best practices for state management in React applications.
Callback Props
In React, callback props serve as a powerful mechanism for enabling communication between child and parent components. By passing functions as props from a parent to a child component, you create a way for the child to interact with and notify the parent about certain events or changes. This fosters dynamic behavior and enhances the overall interactivity of your React application.
6.1 Using Callback Props:
6.1.1 Parent Component:
// ParentComponent.jsx
import React, { useState } from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const [message, setMessage] = useState("");
// Callback function to be passed as a prop
const handleChildClick = (newMessage) => {
setMessage(newMessage);
};
return (
<div>
<p>Message from Child: {message}</p>
<ChildComponent onClick={handleChildClick} />
</div>
);
};
export default ParentComponent;
6.1.2 Child Component:
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ onClick }) => {
const handleClick = () => {
// Call the parent's callback function with a new message
onClick("Hello from Child!");
};
return (
<button onClick={handleClick}>Click me</button>
);
};
export default ChildComponent;
In this example, the ParentComponent
defines a callback function (handleChildClick
) and passes it down to the ChildComponent
as a prop named onClick
. When the button in the ChildComponent
is clicked, it invokes the handleClick
function, triggering the callback passed from the parent. This callback updates the state in the parent component, leading to a dynamic interaction between the parent and child.
6.2 Benefits of Callback Props:
- Dynamic Communication: Callback props facilitate dynamic communication between components, allowing child components to inform their parent components about specific events or changes.
- Encapsulation: Child components can encapsulate their logic and functionality while still influencing the behavior of their parent components through well-defined callback props.
- Reusability: Callback props contribute to the reusability of components by making them more versatile. A single child component can be used in various contexts and customize its behavior based on the provided callback functions.
6.3 Considerations:
- Callback Function Signature: Ensure that the callback function passed as a prop has a well-defined signature, making it clear what parameters the child component should provide when invoking the callback.
- Avoid Excessive Nesting: Be mindful of the depth of callback props, as excessive nesting may lead to prop drilling. Consider using the Context API or state management libraries for more complex scenarios.
By incorporating callback props into your React components, you empower child components to interact with their parent components in a dynamic and controlled manner. This chapter has introduced the concept of callback props and demonstrated their practical usage. In the subsequent chapters, we’ll explore advanced techniques for managing state and communication in React applications.
Best Practices
7.1 Keep Props Immutable
In React, treating props as immutable ensures that the data passed to a component remains unchanged during its lifecycle. This practice contributes to more predictable, bug-free components by preventing unintentional modifications to the props. Immutability encourages a functional programming approach, making components easier to reason about and debug.
Benefits of Immutable Props:
- Predictability: Immutable props guarantee that the data received by a component won’t be altered accidentally, leading to more predictable behavior.
- Debugging: When debugging, knowing that props won’t change simplifies the process of identifying the source of issues within a component.
- Functional Programming: Embracing immutability aligns with functional programming principles, promoting cleaner and more maintainable code.
Immutable Props Example:
// Good practice: Treating props as immutable
import React from 'react';
const ImmutableComponent = ({ name, age }) => {
// No modifications to props within the component
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
};
export default ImmutableComponent;
7.2 Prop Naming Conventions
Adopting consistent and meaningful prop naming conventions enhances code readability and maintainability. Clear and descriptive prop names make it easier for developers to understand the purpose and usage of each prop within a component.
Prop Naming Best Practices:
- Descriptive Names: Use names that clearly convey the purpose of the prop. Avoid generic names like
data
orvalue
unless they accurately represent the content. - Consistency: Maintain a consistent naming style across components. This consistency aids developers in quickly understanding prop conventions within your application.
- Avoid Ambiguity: Choose names that leave little room for ambiguity. If a prop represents a specific entity or action, reflect that in its name.
Prop Naming Conventions Example:
// Good practice: Descriptive and consistent prop names
import React from 'react';
const NamedPropsComponent = ({ firstName, lastName, onClickHandler }) => {
return (
<div onClick={onClickHandler}>
<p>First Name: {firstName}</p>
<p>Last Name: {lastName}</p>
</div>
);
};
export default NamedPropsComponent;
7.3 Avoid Overusing Props
While props are a fundamental mechanism for passing data between components, it’s essential to strike a balance and avoid overusing them. Understanding when to use props and when to manage state within components is crucial for optimal performance and code organization.
Considerations:
- Component Responsibility: A component should primarily focus on rendering UI and managing its local state. Avoid burdening components with too many props, especially if they are not directly related to the component’s responsibility.
- Prop Drilling: Be cautious of excessive prop drilling, where props are passed through multiple levels of components. Evaluate whether the use of context or state management libraries would provide a cleaner solution.
- Performance Impact: Passing large datasets as props might have a performance impact, especially if the data doesn’t change frequently. In such cases, consider alternative solutions, such as fetching data directly within the component.
Optimizing Prop Usage Example:
// Good practice: Balancing props and component responsibility
import React from 'react';
const OptimizedComponent = ({ data }) => {
// Component focuses on rendering and local state management
return (
<div>
{data.map(item => (
<p key={item.id}>{item.name}</p>
))}
</div>
);
};
export default OptimizedComponent;
By following these best practices for props in React, you enhance the maintainability, readability, and performance of your components. Consistently treating props as immutable, adopting clear naming conventions, and judiciously using props contribute to the overall robustness of your React applications. In the subsequent chapters, we’ll continue exploring advanced concepts and techniques for building scalable and efficient React applications.
Advanced Prop Patterns
8.1 Render Props
Render props is a powerful pattern in React that allows components to share code and functionality by passing a function as a prop. This pattern enables the creation of highly flexible and reusable components.
8.1.1 Understanding Render Props:
- Component with Render Prop:
// RenderPropComponent.jsx
import React from 'react';
const RenderPropComponent = ({ render }) => {
// Some component logic or state management...
return (
<div>
{/* Call the render prop function with data */}
{render(data)}
</div>
);
};
export default RenderPropComponent;
Using Component with Render Prop:
// App.js
import React from 'react';
import RenderPropComponent from './RenderPropComponent';
const App = () => {
return (
<div>
{/* Use RenderPropComponent with a render prop function */}
<RenderPropComponent
render={(data) => (
<p>Data from RenderPropComponent: {data}</p>
)}
/>
</div>
);
};
export default App;
Benefits of Render Props:
- Flexibility: Render props allow components to be highly flexible as the rendering logic is provided by the parent component.
- Reusability: Components using render props can be reused in various contexts, adapting their behavior based on different render functions.
- Shareable Logic: Logic and state management in the parent component can be easily shared with the child component using the render prop.
8.2 Higher-Order Components (HOCs)
Higher-Order Components (HOCs) are functions that take a component and return a new component with enhanced functionality. HOCs are a powerful pattern for code reuse and logic sharing across different components.
8.2.1 Creating a Higher-Order Component:
// withEnhancement.jsx
import React from 'react';
const withEnhancement = (WrappedComponent) => {
// Define the logic or additional props to be provided
const enhancedProps = {
additionalProp: "Enhanced Data",
};
// Return a new component with enhanced functionality
return (props) => (
<WrappedComponent {...props} {...enhancedProps} />
);
};
export default withEnhancement;
8.2.2 Using the Higher-Order Component:
// App.js
import React from 'react';
import withEnhancement from './withEnhancement';
const EnhancedComponent = withEnhancement((props) => {
return (
<div>
<p>Original Component</p>
<p>Additional Prop: {props.additionalProp}</p>
</div>
);
});
const App = () => {
return (
<div>
{/* Use the enhanced component */}
<EnhancedComponent />
</div>
);
};
export default App;
Benefits of Higher-Order Components:
- Code Reusability: HOCs promote code reusability by encapsulating common functionality and making it available to multiple components.
- Separation of Concerns: Logic and enhancements are separated from the presentation components, leading to cleaner and more maintainable code.
- Dynamic Composition: HOCs allow components to be composed dynamically, applying different enhancements to the same base component.
In conclusion, both render props and higher-order components are advanced prop patterns in React that contribute to code reusability, flexibility, and maintainability. Understanding when to use each pattern depends on the specific requirements of your application. In the next chapters, we’ll explore more advanced concepts and techniques for building sophisticated React applications.
Interview Questions
Explain the significance of PropTypes in React and how they contribute to code quality.
PropTypes in React are a form of type-checking mechanism, ensuring that the data received through props is of the expected type. By defining PropTypes for your components, you introduce a layer of validation that helps catch potential bugs during development. This not only improves code quality but also enhances the maintainability of your codebase by providing clear documentation on the expected prop types for each component.
How can you handle default values for props in a React component?
Handling default values for props in React can be achieved by using the defaultProps
property. By setting default values for specific props, you ensure that your component gracefully handles cases where the parent component does not provide a value for that prop. This contributes to a more robust and error-resistant component, allowing for smoother user experiences.
What is prop drilling, and how can it be mitigated in a React application?
Prop drilling refers to the process of passing props down through multiple layers of components, even when intermediate components don't need the prop. This can lead to decreased code maintainability. To mitigate prop drilling, developers can use techniques like the Context API, which enables the sharing of values between components without the need to pass them explicitly through every level of the component tree.
Explain the concept of Render Props in React and provide an example of how they can be used.
Render Props is a pattern in React where a component's prop is a function that provides the data to be rendered by that component. This pattern enhances component reusability and allows for more dynamic rendering. An example of Render Props is a DataProvider
component that takes a render prop function and provides data to be rendered by the consuming component. This approach is particularly useful when dealing with components that need to share complex behavior or state.
FAQs
How do you pass props in React, and what is the significance of prop drilling?
Props in React are passed from a parent component to a child component through the use of attributes. Prop drilling refers to the challenge of passing props through multiple levels of nested components. While it's a common pattern, it can lead to code that is harder to maintain. Understanding prop drilling is essential for optimizing data flow in your React applications.
What is the purpose of PropTypes, and how do they contribute to code quality?
PropTypes are a way to enforce the type of data being passed to a component through its props. By defining PropTypes, you can catch bugs early in the development process, enhance code readability, and ensure that components receive the correct data types. This contributes to code quality by providing a form of documentation and reducing runtime errors.
How can you handle default values for props in React components?
Handling default values for props is crucial to ensure that components gracefully handle missing or undefined data. React allows you to specify default values for props using the defaultProps
property. This ensures that even if a component receives undefined props, it can still function correctly without causing errors.
What are some advanced patterns for working with React Props?
Beyond basic prop passing, React offers advanced patterns like render props and Higher-Order Components (HOCs). Render props involve passing a function as a prop to a component, allowing dynamic behavior. HOCs, on the other hand, are functions that take a component and return a new enhanced component, enabling the reuse of logic across different components. Understanding these patterns can take your React development skills to the next level.
Conclusion
In conclusion, our journey through the realm of React props has illuminated the path to creating sophisticated and responsive applications. By mastering the art of prop-passing, understanding prop types, and embracing advanced patterns, you’ve equipped yourself with the tools needed to build elegant and maintainable React components.
As you venture forth in your React endeavors, remember that props serve as the linchpin connecting components and enabling seamless communication. The keyword “props in React” is not merely a technicality but a gateway to a world where your components become dynamic storytellers, each prop adding a layer of functionality and interactivity.
So, go forth with confidence, armed with the knowledge of props in React. As you integrate these concepts into your projects, may your components flourish, and your applications thrive. Happy coding!
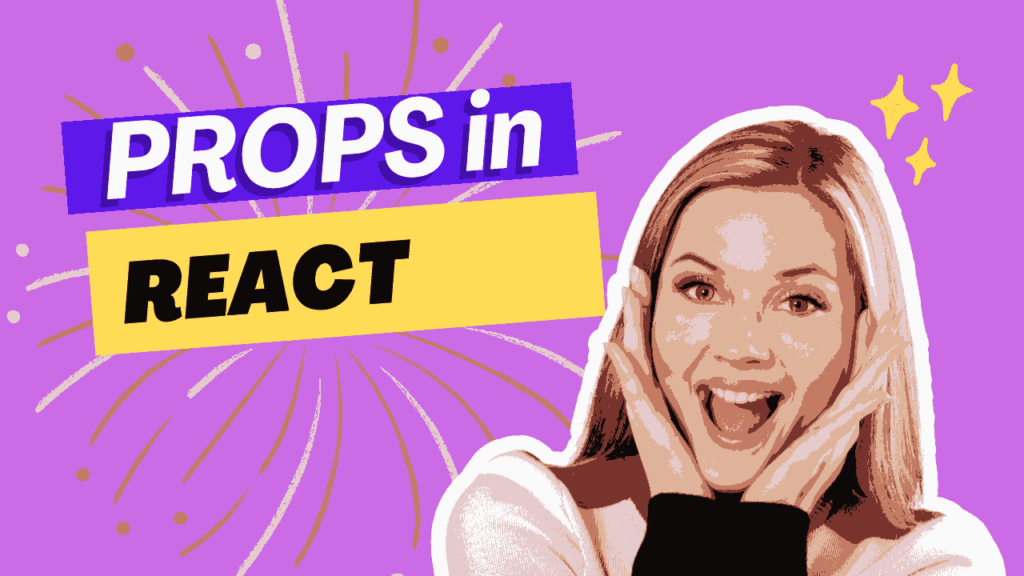