Introduction
Welcome useState
hook to the dynamic world of React development, where crafting interactive and responsive user interfaces is a delightful journey. At the heart of this journey lies a fundamental tool that empowers developers to manage state effortlessly – the useState
hook. In this blog post, we embark on an exploration of the ins and outs of useState
, unraveling its syntax, uncovering its capabilities, and discovering how it serves as the linchpin for building state-of-the-art React applications.
Whether you’re a seasoned developer or just starting with React, understanding the power of useState
is essential. As we delve into this foundational hook, we’ll not only grasp its core concepts but also uncover advanced techniques, best practices, and real-world examples. Get ready to master state management in React and elevate your skills to new heights with the versatile useState
hook.
Table of Contents
useState in React
Understanding State in React
What is State?
In React, “state” refers to the internal data that a component can hold and manage. It represents the current condition or snapshot of the component at any given moment. State allows components to keep track of changing information and enables them to re-render dynamically in response to user interactions, external events, or data updates.
State in React is essentially a JavaScript object that holds key-value pairs. It can store various types of data, such as numbers, strings, booleans, objects, or arrays, depending on the requirements of the component.
The Importance of State in React Applications:
- Dynamic UI: State is fundamental for creating interactive and dynamic user interfaces. It allows components to respond to user input, triggering updates and ensuring that the UI reflects the most recent data.
- Data Persistence: React components are often used to represent parts of the application that need to persist and display changing data. State ensures that these components can manage and display the latest information.
- User Interaction: State is essential for handling user interactions, such as form submissions, button clicks, or any other event that triggers a change in the component’s behavior or appearance.
- Conditional Rendering: Components frequently need to render different content based on certain conditions or user actions. State facilitates conditional rendering by allowing components to adjust their output based on the current data.
- Component Lifecycle: State is closely tied to the lifecycle of React components. As state changes, React automatically re-renders the component, ensuring that the user interface stays up-to-date with the application’s internal state.
- Efficient Updates: React optimizes the updating process by only re-rendering components when their state or props change. This efficiency helps enhance performance, especially in larger applications.
- Single Source of Truth: Using state as the source of truth for a component ensures consistency. Any changes to the component’s data are managed through the state, making it easier to trace and understand how the component behaves over time.
In summary, state is a crucial aspect of React applications, providing a dynamic and responsive structure that allows components to manage and adapt to changing data, ensuring a seamless and engaging user experience.
Introduction to useState
What is useState
?
In React, useState
is a hook that allows functional components to manage state. Prior to the introduction of hooks in React 16.8, state management was primarily done in class components. However, with the advent of hooks, functional components gained the ability to handle state through the useState
hook.
useState
is part of the Hooks API, and it provides a way for components to declare and update state variables. It returns an array with two elements:
- The current state value.
- A function that allows you to update the state.
How to use useState
in Functional Components:
Syntax and Usage:
- Declaration and Initialization: To use
useState
, you typically destructure the returned array into two variables. The first variable holds the current state value, and the second variable is a function used to update the state.
const [state, setState] = useState(initialValue);
Example:
const [count, setCount] = useState(0);
- In this example,
count
is the current state value initialized to 0, andsetCount
is the function used to update the state. - Updating State with the Setter Function: The
setCount
function can be called to update the state. It can take either a new value or a function that calculates the new value based on the previous state.
setCount(count + 1); // Direct value update
Using a functional update (recommended when the new state depends on the current state):
setCount((prevCount) => prevCount + 1);
- The functional update ensures that you are working with the most recent state value, avoiding potential issues in asynchronous updates.
By incorporating useState
in functional components, developers can manage and update state within these components, making them more versatile and capable of handling dynamic data and user interactions.
Syntax and Usage
Declaration and Initialization:
The useState
function is called with an initial value, and it returns an array with two elements. The first element is the current state value, and the second element is a function that can be used to update the state.
const [state, setState] = useState(initialValue);
Example:
const [count, setCount] = useState(0);
In this example, count
is the current state value initialized to 0, and setCount
is the function that will be used to update the state.
Updating State with the Setter Function:
The setCount
function is used to update the state. It can take either a new value or a function that calculates the new value based on the previous state.
Direct Value Update:
setCount(count + 1);
This directly increments the count
state by 1.
Functional Update:
setCount((prevCount) => prevCount + 1);
Using a functional update is recommended when the new state depends on the current state. The prevCount
parameter represents the previous state, and the function returns the new state based on this previous value. This approach ensures that you’re working with the most recent state value, which is crucial for avoiding issues in scenarios involving asynchronous updates.
The syntax and usage of useState
provide a straightforward way to declare, initialize, and update state in functional components, making them more dynamic and responsive to changes in the application’s data or user interactions.
Managing Complex State
Handling Objects and Arrays with useState
:
With useState
, you can manage complex state structures such as objects or arrays by initializing the state with these data types.
Handling an Object:
const [user, setUser] = useState({ name: 'John', age: 25, email: 'john@example.com' });
In this example, the user
state is an object with properties like name
, age
, and email
. You can update individual properties using the setter function (setUser
).
setUser({ ...user, age: 26 });
Handling an Array:
const [items, setItems] = useState(['item1', 'item2']);
For arrays, you can update them similarly. For instance, to add a new item to the array:
setItems([...items, 'newItem']);
Combining Multiple useState
Hooks for Complex State Logic:
When dealing with more complex state logic, it’s common to use multiple useState
hooks to manage different pieces of state independently. This can make your code more modular and easier to understand.
const [name, setName] = useState('John'); const [age, setAge] = useState(25); const [email, setEmail] = useState('john@example.com');
Each piece of state can be managed separately, and updates to one piece of state won’t affect the others.
setName('Jane'); // Updates only the 'name' state setAge(26); // Updates only the 'age' state setEmail('jane@example.com'); // Updates only the 'email' state
This approach is beneficial when dealing with components that have multiple independent aspects of their state. It promotes better organization and readability, as each state variable has its own setter function.
By combining useState
hooks for different aspects of state and handling objects or arrays appropriately, you can effectively manage complex state logic in React functional components.
Functional Updates with useState
Understanding Functional Updates:
In React, functional updates with useState
involve using a function to update the state based on the previous state. This approach is often recommended when the new state depends on the current state. The setState
function provided by useState
allows you to pass a function that receives the previous state as an argument.
Example:
const [count, setCount] = useState(0); // Direct value update setCount(count + 1); // Functional update setCount((prevCount) => prevCount + 1);
In the functional update example, the prevCount
parameter represents the previous state (count
). This ensures that the update is based on the most recent state value, which can be crucial in scenarios involving asynchronous updates or when state changes are closely related.
Use Cases and Benefits:
1. Preventing Race Conditions:
- In scenarios where state updates are asynchronous, using functional updates can prevent race conditions. It ensures that the update is based on the most recent state, reducing the likelihood of unexpected behavior.
2. Dependency on Previous State:
- When the new state value depends on the current state, functional updates provide a concise and reliable way to calculate the new state. This is especially common in scenarios like incrementing a counter or updating a value based on its previous value.
Example:
setCount((prevCount) => prevCount + 1);
3. Avoiding Stale Closures:
- Functional updates help avoid issues related to stale closures. In JavaScript, closures capture the values of variables at the time a function is created. When dealing with asynchronous code, the value of the variable might change before the function is executed. Functional updates ensure the function always gets the latest state value.
4. Ensuring Accurate State Changes:
- Functional updates provide a more reliable way to ensure that state changes are accurate and predictable. This is particularly important when dealing with complex state logic or when state updates are triggered by user interactions.
5. Consistent Behavior Across Renders:
- Functional updates help maintain consistent behavior across multiple renders. Since the function receives the previous state, it allows React to apply updates consistently, even if there are multiple updates within the same render cycle.
In summary, functional updates with useState
are beneficial in scenarios where the new state depends on the current state. They provide a reliable way to handle state updates, prevent race conditions, and ensure accurate and consistent behavior in React functional components.
Effect of Dependencies on useState
Dealing with Asynchronous State Updates:
When working with asynchronous operations that update the state in a React component, it’s crucial to understand how React handles state updates and how to prevent unintended side effects.
Example with Asynchronous Update:
const handleAsyncUpdate = () => { // Assume some asynchronous operation fetchData().then((data) => { // This state update may not behave as expected! setCount(count + 1); }); };
In this example, if fetchData()
takes some time to complete, there’s a chance that count
is not the current state value when the update is applied. This can lead to unexpected behavior.
Dependency Array and Its Role in Preventing Side Effects:
The dependency array in the useEffect
hook plays a significant role in preventing side effects caused by asynchronous state updates.
Example using useEffect
with Dependency Array:
useEffect(() => { fetchData().then((data) => { // Using the functional update to ensure the correct previous state setCount((prevCount) => prevCount + 1); }); }, [/* dependencies go here */]);
Dependency Array:
- By providing an empty dependency array (
[]
), you ensure that theuseEffect
callback runs once when the component mounts. - However, if there are dependencies (variables) inside the array, the
useEffect
callback will run whenever any of these dependencies change.
Preventing Side Effects:
- Including the relevant dependencies in the array ensures that the
setCount
function inside theuseEffect
callback operates on the most up-to-date values. - This prevents side effects caused by asynchronous operations finishing at unexpected times.
Full Example:
const MyComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const handleAsyncUpdate = async () => {
const data = await fetchData();
setCount((prevCount) => prevCount + 1);
};
handleAsyncUpdate();
}, [/* dependencies go here */]);
return (
<div>
<p>Count: {count}</p>
</div>
);
};
In this example, if fetchData
is an asynchronous function, using the dependency array ensures that setCount
operates on the correct previous state, preventing potential side effects and ensuring a predictable behavior. It’s essential to include all dependencies in the array that are used inside the useEffect
callback to maintain consistency.
Best Practices and Tips
When to Use useState
vs Other State Management Solutions:
- Use
useState
When Local Component State is Sufficient:- For managing state within a specific component, especially if the state is not shared with other components,
useState
is a suitable and straightforward choice.
- For managing state within a specific component, especially if the state is not shared with other components,
- Consider Other Solutions for Global State:
- If the state needs to be shared among multiple components or if the application state is complex, consider using solutions like Redux, Context API, or Recoil for managing global state.
Naming Conventions and Code Organization:
- Descriptive Variable Names:
- Use clear and descriptive names for state variables to enhance code readability. Avoid generic names like
data
orvalue
.
- Use clear and descriptive names for state variables to enhance code readability. Avoid generic names like
- Consistent Naming Conventions:
- Establish consistent naming conventions for state variables across your codebase. This improves maintainability and reduces confusion.
- Organize Code Logically:
- Group related logic together, and consider breaking down complex components into smaller, more manageable ones. This makes the codebase easier to understand and maintain.
Real-world Examples
Building a Simple Counter Application:
import React, { useState } from 'react';
const CounterApp = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default CounterApp;
Implementing a Form with Controlled Components
import React, { useState } from 'react';
const FormApp = () => {
const [formData, setFormData] = useState({
username: '',
password: '',
});
const handleInputChange = (e) => {
setFormData({
...formData,
[e.target.name]: e.target.value,
});
};
const handleSubmit = (e) => {
e.preventDefault();
// Process form data
console.log('Form submitted:', formData);
};
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input
type="text"
name="username"
value={formData.username}
onChange={handleInputChange}
/>
</label>
<label>
Password:
<input
type="password"
name="password"
value={formData.password}
onChange={handleInputChange}
/>
</label>
<button type="submit">Submit</button>
</form>
);
};
export default FormApp;
Advanced Techniques
Custom Hooks that Leverage useState
:
import { useState } from 'react';
const useToggle = (initialValue = false) => {
const [state, setState] = useState(initialValue);
const toggle = () => {
setState(!state);
};
return [state, toggle];
};
// Usage
const MyComponent = () => {
const [isToggled, toggle] = useToggle(false);
return (
<div>
<p>Is Toggled: {isToggled ? 'Yes' : 'No'}</p>
<button onClick={toggle}>Toggle</button>
</div>
);
};
Handling Side Effects with useEffect
in Conjunction with useState
import { useState, useEffect } from 'react';
const SideEffectExample = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data asynchronously
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []); // Empty dependency array to run the effect only once
return (
<div>
{data ? (
<p>Data: {data}</p>
) : (
<p>Loading...</p>
)}
</div>
);
};
Common Pitfalls and How to Avoid Them
Accidental Closures and Their Impact
import React, { useState } from 'react';
const ClosureExample = () => {
const [count, setCount] = useState(0);
const increment = () => {
// Incorrect: This will capture the initial count value
setTimeout(() => {
setCount(count + 1);
}, 1000);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment after 1 second</button>
</div>
);
};
export default ClosureExample;
Solution: Use the functional update form to avoid capturing stale state.
const increment = () => {
// Correct: Using functional update to capture the latest count value
setTimeout(() => {
setCount((prevCount) => prevCount + 1);
}, 1000);
};
Misusing Functional Updates
const MisuseExample = () => {
const [count, setCount] = useState(0);
const incorrectUpdate = () => {
// Incorrect: Misusing functional update, no need for a function here
setCount(count + 1);
};
const correctUpdate = () => {
// Correct: Using a functional update when the new state depends on the previous state
setCount((prevCount) => prevCount + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={incorrectUpdate}>Incorrect Update</button>
<button onClick={correctUpdate}>Correct Update</button>
</div>
);
};
export default MisuseExample;
Solution: Use functional updates when the new state depends on the previous state. For non-dependent updates, direct value updates are appropriate.
setCount((prevCount) => prevCount + 1); // Correct for dependent updates
setCount(count + 1); // Correct for non-dependent updates
Advantages
`
useState
in React is a fundamental and versatile hook that provides a simple way to manage state in functional components. Its advantages make it an essential tool for React developers:
- Simplicity and Readability:
useState
simplifies state management in functional components by eliminating the need for class components and making state logic more concise and readable.
- Ease of Use:
- The syntax for using
useState
is straightforward. It involves declaring a state variable and its updater function, which makes it easy for developers to understand and implement.
- The syntax for using
- Lightweight and Performant:
useState
is lightweight compared to class components, contributing to better performance. It allows React to optimize and avoid unnecessary re-renders by updating only the components that depend on the changed state.
- Functional Updates:
useState
supports functional updates, allowing you to update the state based on the previous state. This helps in scenarios where state updates depend on the current state, preventing race conditions and ensuring data consistency.
- Multiple States in a Component:
- You can use multiple
useState
hooks in a single component, enabling you to manage different pieces of state independently. This modularity enhances code organization and maintainability.
- You can use multiple
- Integration with Hooks Ecosystem:
useState
seamlessly integrates with other React hooks, such asuseEffect
and custom hooks. This cohesive ecosystem simplifies the development process and encourages the use of modular and reusable code.
- Compatibility with Functional Paradigm:
useState
aligns with the functional programming paradigm, allowing developers to embrace a more declarative and immutable approach to state management. This promotes better code predictability and reduces side effects.
- Ideal for Local Component State:
- For managing local component state (as opposed to global state),
useState
is the preferred choice. It keeps the state confined to the component, preventing unnecessary complexity in larger applications.
- For managing local component state (as opposed to global state),
- Enhanced Testing:
useState
contributes to improved testability as it allows for easy isolation and testing of specific components. This makes it simpler to write unit tests for individual components without the need for complex setup.
- Accessibility to Lifecycle Methods:
- With
useState
, functional components gain the ability to manage component-level state, blurring the line between functional and class components. This accessibility simplifies the transition for developers familiar with class component lifecycle methods.
- With
In summary, the useState
hook in React provides a clean, efficient, and flexible solution for managing state in functional components, making it an indispensable tool for building modern and scalable React applications.
Current trends and developments
- Concurrent Mode and
useState
Enhancements: React’s Concurrent Mode is an experimental set of features aimed at making React apps more responsive. There may be enhancements or optimizations related touseState
in the context of Concurrent Mode. - React Hooks Adoption: The adoption of React Hooks, including
useState
, has likely continued to grow, with developers preferring functional components and hooks over class components for state management. - New Features or Syntax Improvements: React evolves, and new features or syntax improvements are periodically introduced. Keep an eye out for any new additions to the React library that might affect the usage or capabilities of
useState
. - State Management Libraries and Alternatives: While
useState
is a fundamental part of React, developers often explore additional state management solutions for more complex applications. Check if there are emerging libraries or patterns that work alongside or as alternatives touseState
. - Integration with Concurrent React Libraries: As Concurrent React progresses, libraries and tools supporting concurrent rendering may provide additional functionality or optimizations that work in conjunction with
useState
. - Best Practices and Patterns: The React community continually refines best practices and patterns for using
useState
. Keep an eye on discussions around efficient state management, avoiding common pitfalls, and optimizing performance. - Tooling Support: Improved tooling support for React, including linters, IDE extensions, and debugging tools, might have emerged to enhance the developer experience when working with
useState
.
To stay updated on the latest trends and developments, it’s recommended to regularly check the official React blog, GitHub repository, and participate in the React community through forums like Stack Overflow or Reactiflux Discord. Additionally, explore articles, tutorials, and conference talks for insights into how developers are using and evolving state management in React.
Future Outlooks
- Continued Emphasis on Functional Components: React has been moving towards a more functional component-centric paradigm with the introduction of hooks. The use of
useState
is likely to persist and even become more central to React development as functional components continue to be the primary building blocks. - Enhancements and Additions: React is an actively developed library, and there may be enhancements or additions to
useState
to provide more features or options. Developers might see improvements related to performance, concurrent rendering, or additional capabilities for managing complex state. - Integration with Concurrent Mode: As Concurrent Mode progresses and becomes more stable,
useState
may see further integration or enhancements to leverage concurrent rendering features, improving the performance and responsiveness of React applications. - Tooling and DevTools Support: React’s ecosystem includes robust tools and DevTools for developers. Future developments may include improved tooling support for
useState
, making it easier for developers to debug, profile, and optimize their applications. - Ecosystem Evolution: The React ecosystem is dynamic, and developments in state management libraries or patterns might influence how developers approach state in React applications. While
useState
will remain fundamental, there could be emerging best practices or alternative solutions for specific use cases. - Global State Management: While
useState
is excellent for managing local component state, developers often turn to additional state management solutions for global state. Future developments might explore more streamlined ways to manage global state within the React core or through endorsed patterns. - React Concurrent UI Patterns: The React team has been working on Concurrent UI patterns, which may impact how state is managed in various parts of an application. Keep an eye on evolving UI patterns and guidelines that emerge as Concurrent Mode matures.
- Community Feedback and Contributions: React development heavily relies on community feedback. The future of
useState
will likely be influenced by the experiences and suggestions of the React developer community. Follow discussions on GitHub, forums, and other community platforms to stay informed about potential changes.
Remember to regularly check the official React documentation, blog, and GitHub repository for the latest updates. React’s commitment to backward compatibility ensures a smooth transition for developers, but staying informed about new features and best practices is key to harnessing the full potential of React and useState
.
Interview Questions
How can you use multiple state variables with useState in a single component?
You can use useState
multiple times to manage different state variables in a single component. Each call to useState
returns a separate state variable and its corresponding updater function. Example:
import React, { useState } from 'react';
function UserProfile() {
const [username, setUsername] = useState('');
const [email, setEmail] = useState('');
// Rest of the component logic
}
Explain the concept of functional updates with useState. When and why would you use them?
Functional updates involve passing a function to setState
that receives the previous state and returns the new state. This is useful when the new state depends on the previous state to avoid race conditions. Example:
const [count, setCount] = useState(0);
// Using functional update
const increment = () => setCount((prevCount) => prevCount + 1);
Can you describe a scenario where using useState might not be the best choice for state management?
useState
is ideal for managing local component state. However, for complex applications with shared state between multiple components, or when state needs to be persisted across different routes, using a state management library like Redux or context API might be more appropriate.
How does the dependency array in useEffect relate to state variables managed by useState?
The dependency array in useEffect
specifies which values, when changed, should trigger the effect to run. If a state variable managed by useState
is used inside the useEffect
, it should be included in the dependency array to ensure the effect runs when that state changes. Failing to include it may lead to stale closures and unintended behavior. Example:
useEffect(() => {
// Effect logic
}, [count]); // Include 'count' in the dependency array
FAQ’s
Can I use multiple useState hooks in a single component?
Yes, you can use multiple useState
hooks in a single component. This allows you to manage different pieces of state independently. Each useState
call initializes a separate state variable and its corresponding updater function.
const [name, setName] = useState('John');
const [age, setAge] = useState(25);
How do I update state based on the previous state with useState?
To update state based on the previous state, use the functional update form of setState
. The updater function receives the previous state as an argument, and you can return the new state based on it.
setCount((prevCount) => prevCount + 1);
What are the common mistakes to avoid when using useState?
- Forgetting to call
useState
with the initial state. - Not using the functional update form when the new state depends on the previous state.
- Incorrectly updating objects or arrays directly (use the spread operator or other immutability techniques).
- Assuming state updates are synchronous (they are asynchronous).
- Misusing
useState
in conditions or loops.
When should I use useState versus other state management solutions in React?
useState
is suitable for managing local component state. Use it when:
- The state is specific to a component and doesn't need to be shared globally.
- You want to embrace a more functional programming paradigm.
- Your component doesn't require complex state management.
For global state, consider using tools like React Context API or state management libraries like Redux for more centralized control.
Conclusion
In the ever-evolving landscape of React development, proficiency in state management is non-negotiable. The useState
hook, our guiding star throughout this exploration, emerges as a key ally in creating applications that seamlessly adapt to user interactions. By now, you’ve not only gained a comprehensive understanding of the useState
syntax and usage but also unearthed advanced techniques and best practices.
As you embark on your React endeavors, armed with the knowledge from this blog post, remember that useState
is more than just a hook; it’s a gateway to crafting immersive user experiences. Whether you’re building a simple counter or tackling complex state logic, let useState
be your trusted companion. May your React journey be filled with dynamic components, responsive interfaces, and the joy of mastering state with the formidable useState
hook. Happy coding!
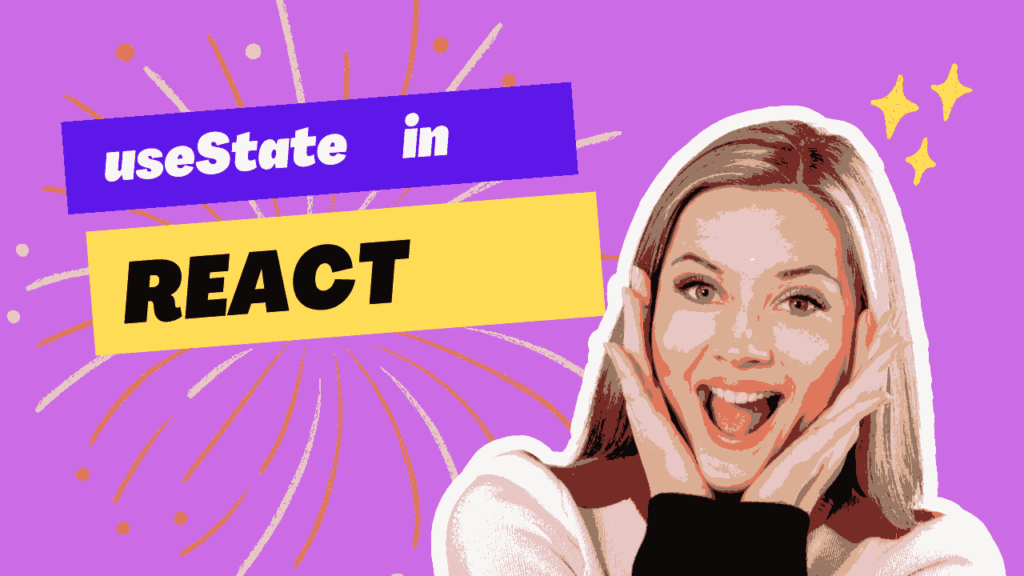